JavaScript Fundamentals: DOM
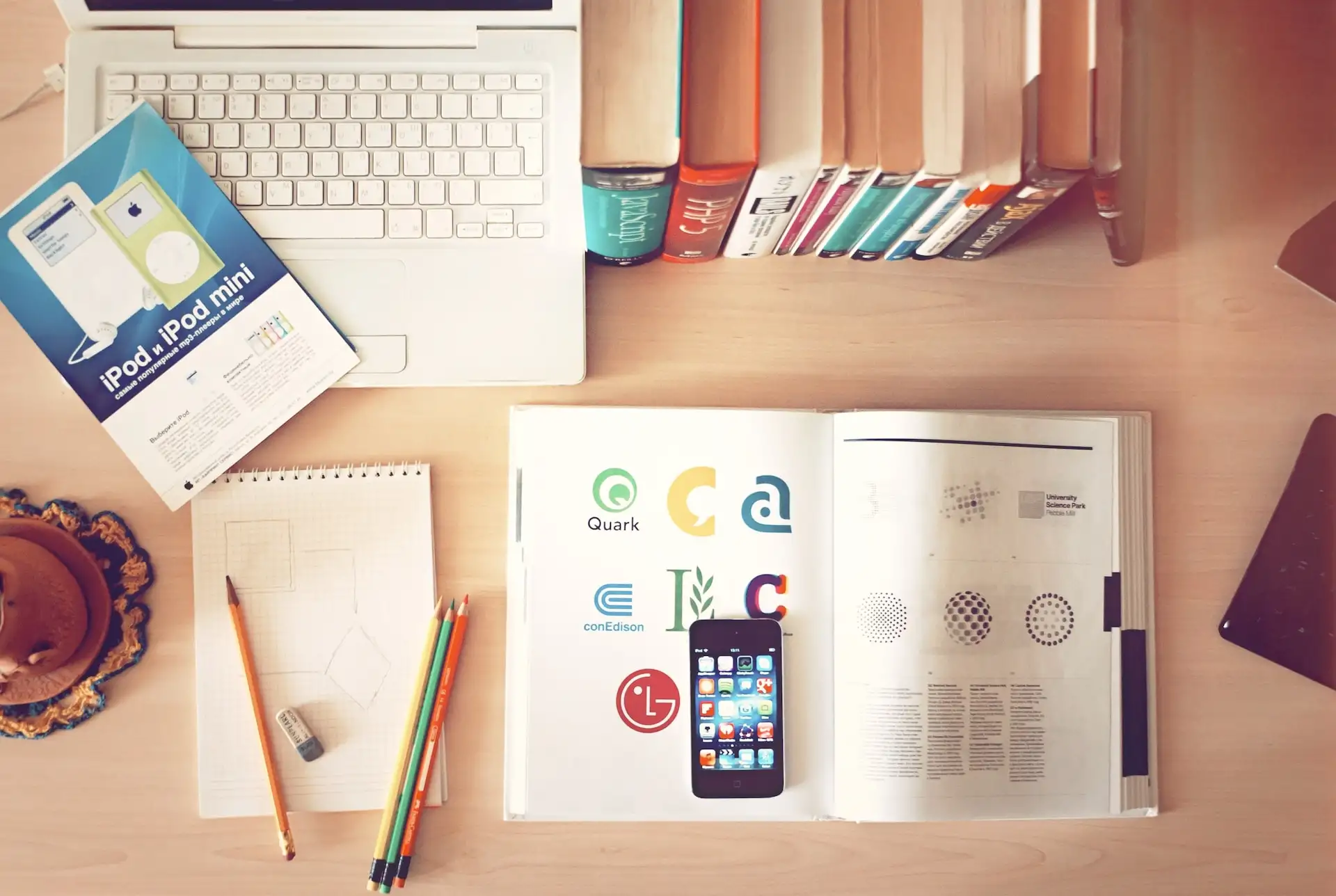
An overview of the DOM:
Dom, Dom, Dom...
What is the DOM? The DOM (The Document Object Model) is a programming interface for web documents. It represents the page so that programs can change the document structure, style, and content. The DOM represents the document as nodes and objects, which allows programming languages to interact with the page.
When a web page is loaded, the browser creates a Document Object Model of the page.
The HTML DOM is a standard object model and programming interface for HTML. It defines:
- The HTML elements as objects
- The properties of all HTML elements
- The methods to access all HTML elements
- The events for all HTML elements
The HTML DOM model is constructed as a tree of Objects:
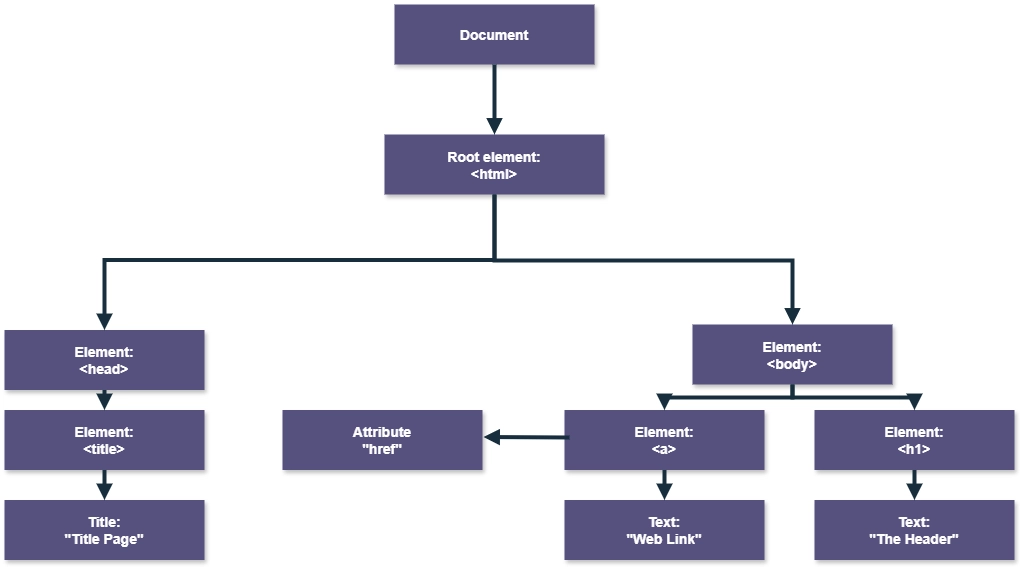
The 4 main types of nodes in the DOM:
- Text nodes
- Element nodes
- Comment nodes
- WhiteSpace nodes
DOM Navigation
Let's have a look at Navigation Properties, which are useful for when elements are close to each other.
Parent Node
-
parentNode : Returns the
parent node
of an element. -
parentElement : Returns the
parent element node
of an element.
Child Nodes
-
childNodes : Returns a collection of child nodes of an element.
-
firstChild : Returns the
first child
node of an element. -
lastChild : Returns the
last child
node of an element. -
children : Returns a collection of
child element nodes
of an element. -
firstElementChild : Returns the
first child element
node of an element. -
lastElementChild : Returns the
last child element
node of an element.
Sibling Nodes
-
nextSibling : Returns the
next sibling
node of an element. -
previousSibling : Returns the
previous sibling
element node of an element. -
nextSibling : Returns the
next sibling
element node of an element. -
previousElementSibling : Returns the
previous element sibling
element node of an element.
Search Elements in DOM
-
getElementById : Gets an element with a specified ID.
-
getElementsByClassName : Gets a collection of elements with a given class name.
-
getElementsByName : Gets a collection of elements with a specified name attribute.
-
getElementsByTagName : Gets a collection of elements with a given tag.
Descendant Nodes
-
querySelectorAll(selector) : Returns a collection of elements that matches a given CSS selector.
-
querySelector(selector) : Returns the
first element
that matches a given CSS selector.
Ancestors
- closest(selector) : Returns the
closest
ancestor element that matches a given CSS selector.