JavaScript Fundamentals: Event Handling
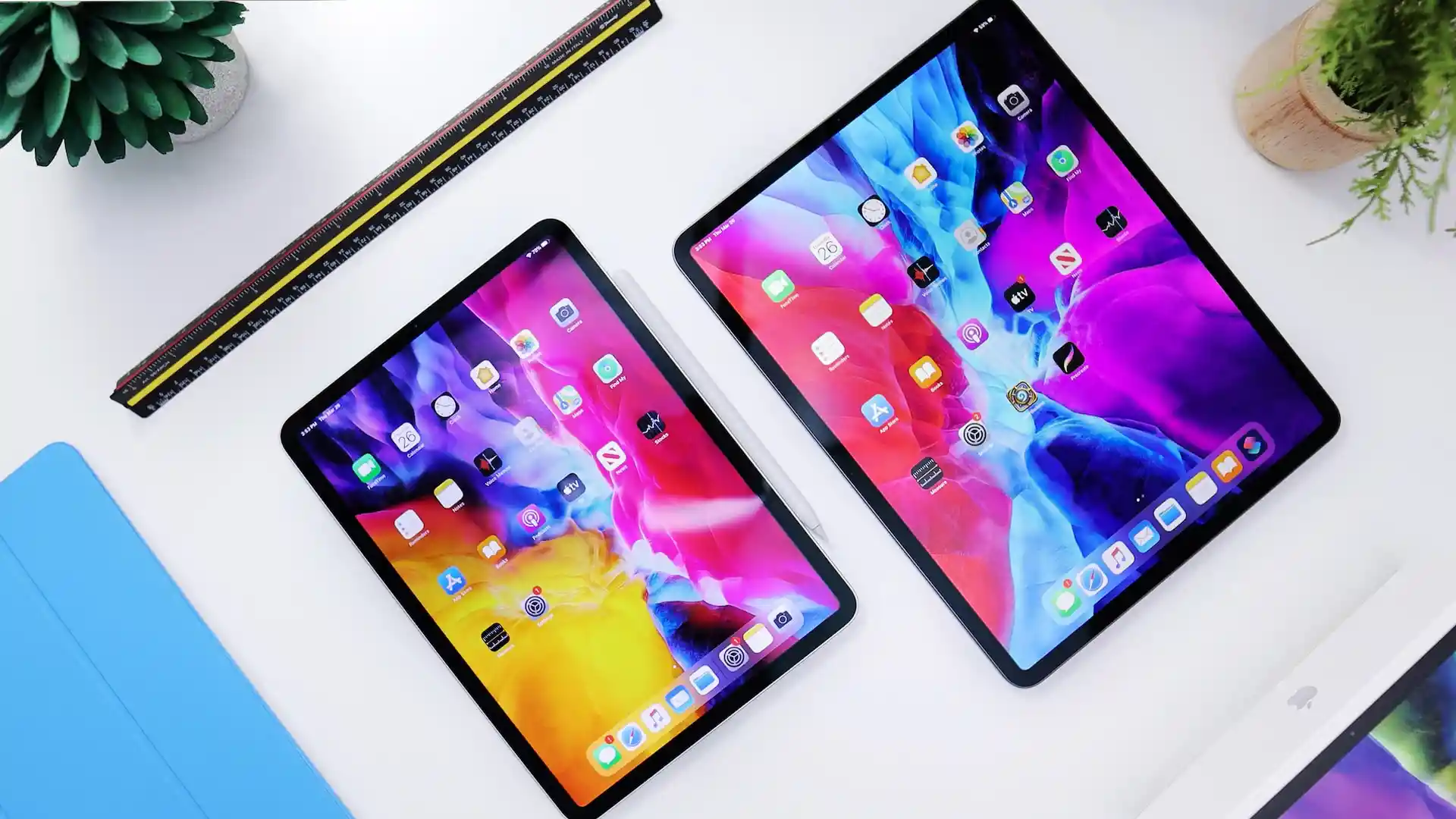
Table Of Content
Let's identify and review examples of different Event Handling features available within JavaScript.
Event Handling
addEventListener
This is the preferred method for attaching event handlers. It allows multiple event listeners and avoids overwriting existing handlers.
button.addEventListener('click', () => {
console.log('Button was clicked!');
});
onclick
Assigns a function directly to the onclick property of an element. Be careful, as it can overwrite existing click handlers.
button.onclick = () => {
console.log('Button was clicked!');
};
onmouseover/onmouseout
Handles mouseover and mouseout events, respectively.
element.onmouseover = () => {
element.style.background = 'white';
};
element.onmouseout = () => {
element.style.background = 'black';
};
onkeydown/onkeyup
Manages keydown (detects when a key is pressed) and keyup (detects when a key is released) events, respectively.
input.onkeydown = (event) => {
if (event.key === 'Enter') {
console.log('Enter key was pressed!');
}
};
input.onkeyup = (event) => {
if (event.key === 'Enter') {
console.log('Enter key was released!');
}
};
onsubmit
Handles the submit event of a Form element. It is good practice to use preventDefault()
because it tells the user agent that if the event does not get explicitly handled, its default action should not be taken.
form.onsubmit = (event) => {
event.preventDefault();
console.log('Form was submitted!');
};
onchange
Responds to changes in the value of an Input element.
input.onchange = () => {
console.log('Input value was changed!');
};
onload
Handles the load event of a window or an image element.
window.onload = () => {
console.log('Window loaded!');
};