JavaScript Fundamentals: Functions
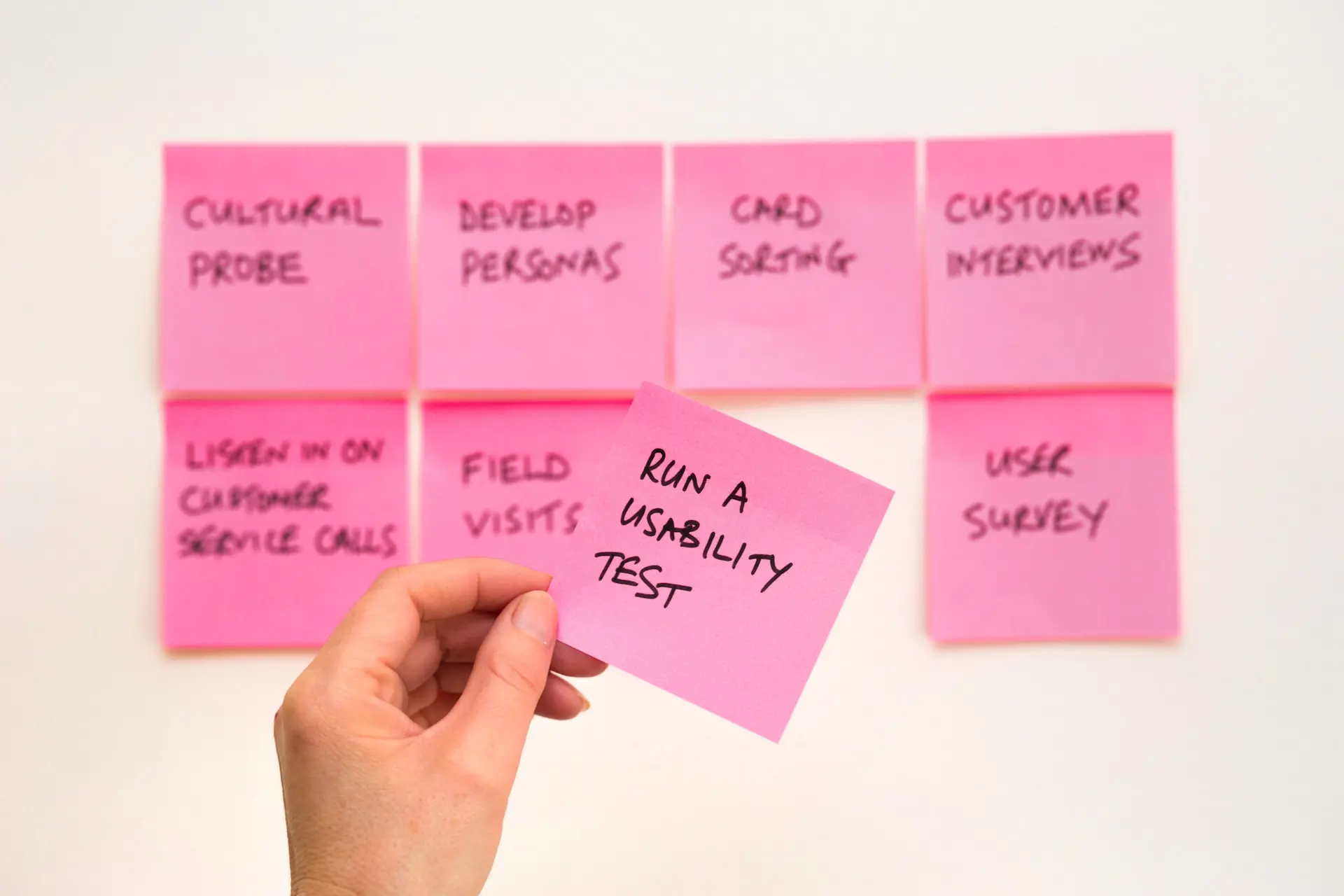
Table Of Content
Let's look at a brief overview of 6 Function types:
Functions
Named Functions - The traditional method.
function myFunction() {
console.log('Hello World!');
}
myFunction();
Anonymous Functions - Functions without a name, are used as function expressions or for arguments.
let greeting = function (name) {
console.log(`Hello ${name}`);
};
greeting('Dan');
// Output: Hello Dan
Arrow Functions - Introduced in the ES6 JS update, allows shorter syntax and one-line functions.
let greeting = () => console.log('Hello');
greeting();
// Output: Hello
IIFE Functions - Immediately Invoked Function Expressions (IIFE), are immediately executed after being called. IIFE are used to create private scopes, and to avoid polluting the global namespace.
(function () {
let string = 'Hi there!';
console.log(string);
})();
// Immediately Invoked Output: Hi there!
Higher Order Functions - Functions that take one or more functions as arguments, or returns a function. Example: map()
, filter()
, reduce()
let array = [5, 10, 15];
let newArray = array.map((element) => element + 10);
console.log(newArray);
// Output: [25, 30, 35]
Constructor Functions - These are used as templates/blueprints for creating objects with similar properties and methods. They are invoked using the new keyword to create instances of objects.
function Person(name, country) {
this.name = name;
this.country = country;
}
let user1 = new Person('Dan', 'Japan');
console.log(
`Hello there! I'm ${user1.name}, and I'm located in ${user1.country}!`
);
// Output: Hello there! I'm Dan, and I'm located in Japan!