javascript
JavaScript Fundamentals: Loop Types
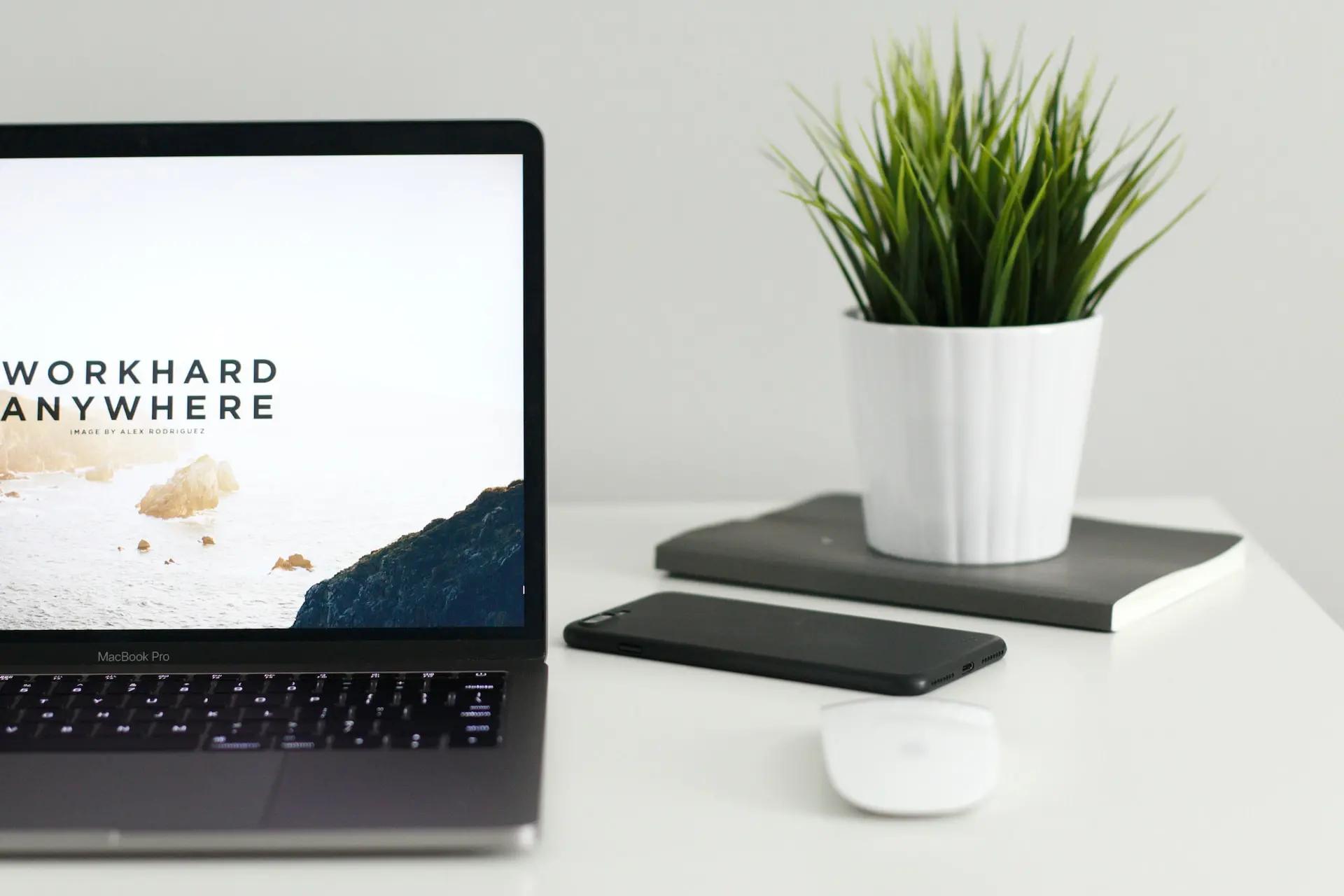
Table Of Content
In this post, we will quickly run through 7 different ways of performing Loops in JavaScript by looking at examples.
Looping
for loop
for (initialExpression; condition; updateExpression) {
// for loop body
}
const cars = ['Toyota', 'Nissan', 'Mitsubishi'];
for (let i = 0; i < cars.length; i++) {
console.log(cars[i]);
}
while loop
while (condition) {
// while loop body
}
const cars = ['Toyota', 'Nissan', 'Mitsubishi'];
let i = 0;
while (i < cars.length) {
console.log(cars[i]);
i++;
}
do-while loop
do {
// do-while loop body
} while (conditon);
const cars = ['Toyota', 'Nissan', 'Mitsubishi'];
let i = 0;
do {
console.log(cars[i]);
i++;
} while (i < cars.length);
forEach loop
array.forEach(function(currentValue, index, arr));
const cars = ['Toyota', 'Nissan', 'Mitsubishi'];
cars.forEach((element) => {
console.log(element);
});
map loop
array.map(function(currentValue, index, arr), thisValue);
const cars = ['Toyota', 'Nissan', 'Mitsubishi'];
const newCars = cars.map((e) => {
return e.toUpperCase();
});
for-of loop
for (element of iterable) {
// for-of loop body
}
const cars = ['Toyota', 'Nissan', 'Mitsubishi'];
for (let element of cars) {
console.log(element);
}
for-in loop
for (key in object) {
// for-in loop body
}
const cars = { 1: 'Toyota', 2: 'Nissan', 3: 'Mitsubishi' };
for (let key in cars) {
console.log(cars[key]);
}