JavaScript Fundamentals: Object Methods
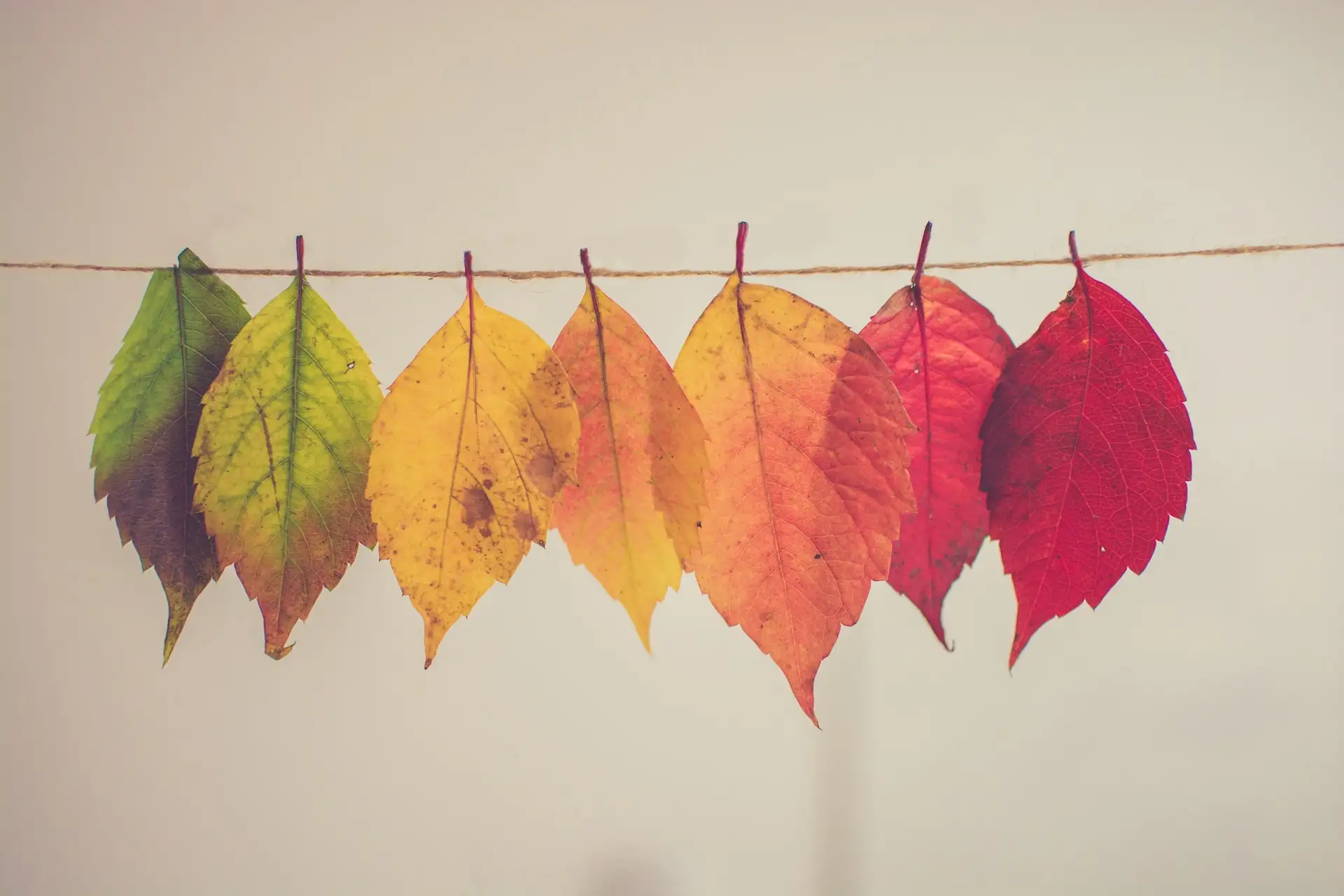
Table Of Content
This is a brief guide of 8 different Object Methods within JavaScript:
Object Methods
keys
This method retrieves all the names of enumerable properties from an object.
It is very useful for situations when it is necessary to iterate over properties, or for when you want an overview of an object's structure.
const car = { brand: 'Toyota', model: 'Yaris' };
console.log(Object.keys(car));
// Output: ['brand', 'model']
values
values is similar to Object.keys(), but returns the actual values instead of the keys.
This is useful when you want the stored data as opposed to the property name.
const car = { brand: 'Toyota', model: 'Yaris' };
console.log(Object.values(car));
// Output: ['Toyota', 'Yaris']
entries
This method combines both keys and values, and produces an array of pairs.
Each pair is represented by an array, and consists of a property name plus its corresponding value.
It is particularly useful when working with functions that expect key-value pairs.
const car = { brand: 'Toyota', model: 'Yaris' };
console.log(Object.entries(car));
// Output: [['brand', 'Toyota',] ['model', 'Yaris']]
assign
This method can copy values from one or multiple source objects to a target object.
This is beneficial if you need to either merge objects, or create copies with additional properties.
const car = { brand: 'Toyota', model: 'Yaris' };
const color = { color: 'blue' };
const carWithColor = Object.assign(car, color);
console.log(carWithColor);
// Output: {brand: 'Toyota', model: 'Yaris', color: 'blue' }
freeze
When the freeze method is used, you cannot add, delete, or modify any of its properties. This is important if you want to ensure data integrity, and prevent additional manipulation.
const car = { brand: 'Toyota', model: 'Yaris' };
car.year = 2024;
// Output: Error
is
This checks if 2 values are the same, including positive and negative zero-value.
console.log(Object.is('hello', 'hello')); // Output: true
console.log(Object.is(NaN, NaN));
// Output: true
// NaN === NaN will return false
defineProperty
This is an advanced tool for adding new properties, or modify existing properties.
You can set characteristics, including: enumerability, writability, and configurability for the property. As such, this method offers highly detailed control over a property.
const car = { brand: 'Toyota', model: 'Yaris' };
Object.defineProperty(car, 'year', { value: 2024, writable: false });
console.log(car.year);
// Output: 2024
hasOwnProperty
This method checks if an object has a specific property as its direct property. That is, not inherited from its prototype.
This is a better solution to check for properties than using the in oeprator, especially when working with objects that might have modified built-in properties.
const car = { brand: 'Toyota', model: 'Yaris' };
console.log(car.hasOwnProperty('brand));
// Output: true