JavaScript Fundamentals: Promises
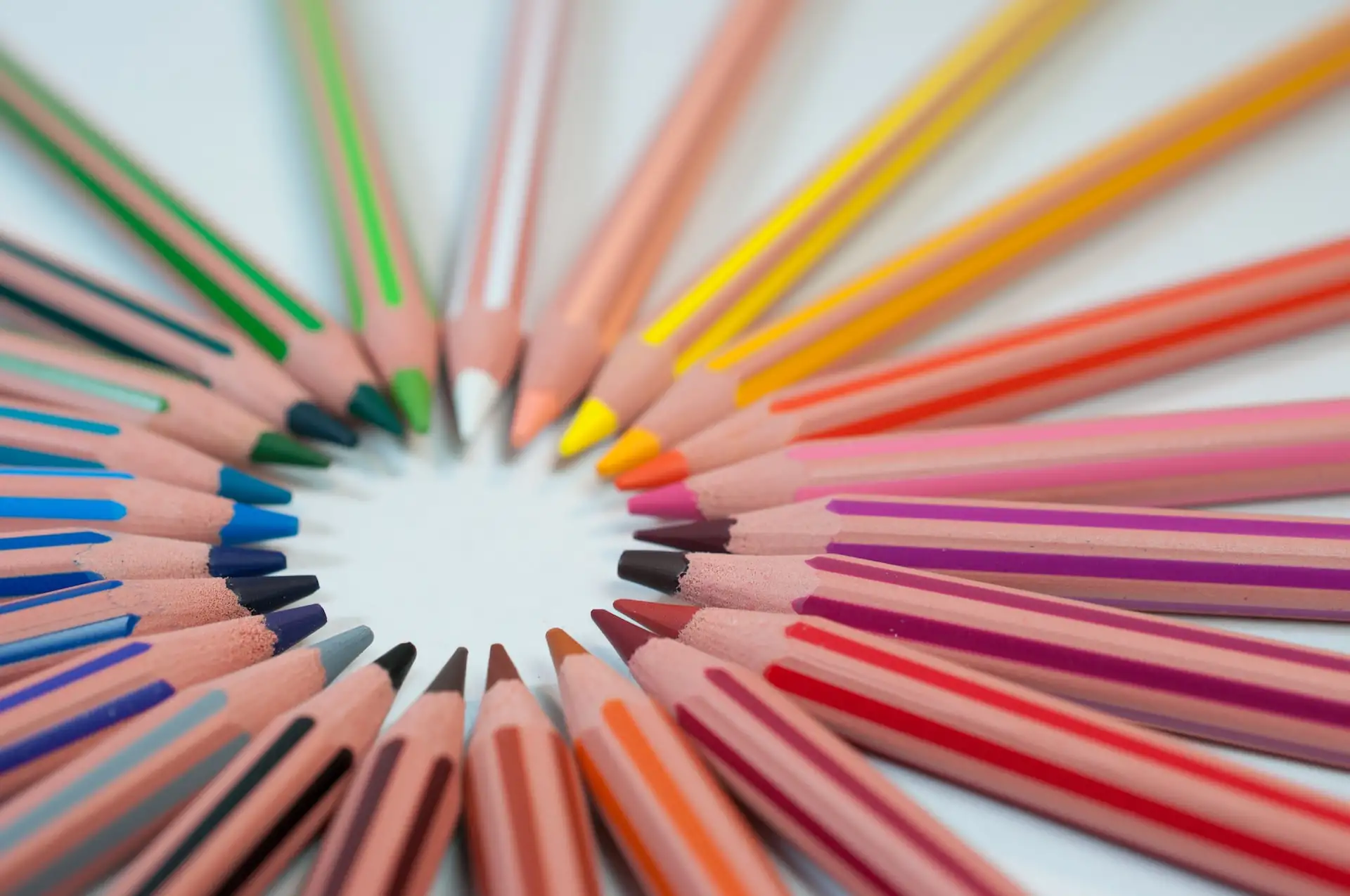
Table Of Content
Promises
A Promise is a good way to handle asynchronous operations, and is used to identify if an asynchronous operation was successfully completed or not. Basically, a Promise can be thought of as a container for a future value.
A Promise can have 1 of 3 states:
- Pending - a process is not complete
- Fulfilled - the operation was a success
- Rejected - an error occurred
One of the main benefits of Promises is that we no longer need to rely on events and callbacks passed into asynchronous functions to handle asynchronous results. So instead of nesting callbacks, we can chain promises for a sequence of asynchronous operations, which allows us to escape from callback hell.
Create a Promise:
To create a Promise object, we use the Promise()
constructor.
let promise = new Promise(function(resolve, reject)) {
// action
}
Example:
const count = true;
let countValue = new Promise(function (resolve, reject) {
if (count) {
resolve('There is a count value.');
} else {
reject('There is no count value.');
}
});
Promise Chaining:
Promises are useful when you have to handle multiple asynchronous tasks, one after another.
You can peform an operation after a Promise is resolved using methods: then()
, catch()
and finally()
.
let promise = new Promise(function(resolve, reject)) {
// action
}
then() method:
The then()
method is used with the callback when the promise is either successfully fulfilled or resolved.
You can chain multiple then()
methods with the promise.
// return a promise
let countValue = new Promise(function (resolve, reject) {
resolve('Promise was resolved.');
});
//executes when promise is resolved successfully
countValue
.then(function successValue(result) {
console.log(result);
})
.then(function successValue2() {
console.log('You can call multiple functions using this.');
});
catch() method:
The catch()
method is used with the callback when the promise is rejected or if an error happens.
// return a promise
let countValue = new Promise(function (resolve, reject) {
reject('Promise was rejected.');
});
//executes when promise is resolved successfully
countValue
.then(function successValue(result) {
console.log(result);
})
.catch(
// executes if an error happens
function errorValue(result) {
console.log(result);
}
);
// Promise was rejected
finally() method:
The finally()
method is executed when the promise is either successfully resolved or rejected.
You can chain multiple then()
methods with the promise.
// return a promise
let countValue = new Promise(function (resolve, reject) {
resolve('Promise resolved.');
});
// add other blocks of code
countValue.finally(function hello() {
console.log('This code was executed.');
});
Promises vs. Callback:
Promises
- Easy to read and user-friendly syntax.
- Error handling is easier to manage.
api()
.then(function (result) {
return api2();
})
.then(function (result2) {
return api3();
})
.then(function (result3) {
// execute code
})
.catch(function (error) {
// handle errors that might occur
});
Callbacks
- Difficult to follow syntax.
- Error handling could be difficult to manage.
- May lead to the dreaded callback hell (a callback is called insider another callback, and so on).
api(function (result) {
api2(function (result2) {
api3(function (result3) {
// execute code
if (error) {
// take action
} else {
// take action
}
});
});
});