JavaScript Advanced: Scopes & Type Coercion
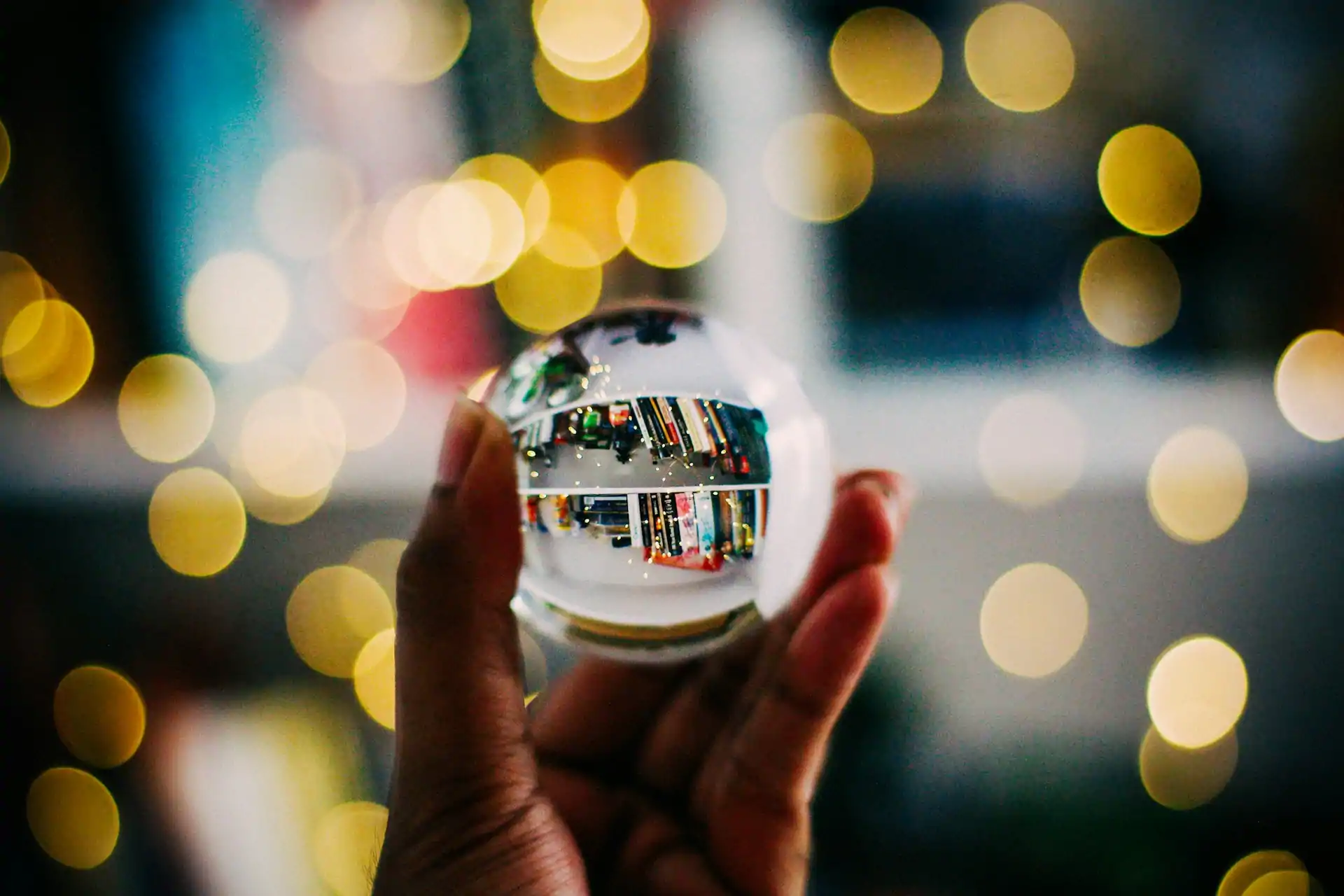
Table Of Content
We will simplify 2 challenging advanced JavaScript topics: Scopes & Type Coercion.
Scopes
Scoping: How a program's variables are organized and accessed.
Lexical environment: the scope or environment the engine is currently reading code in.
A new lexical environment is created when curly brackets are used, even nested brackets {{...}}
create a new lexical environment.
The execution context tells the engine which lexical environment it is currently working in and the lexical scope determines the available variables.
Scope: The environment in which a certain variable is declared. There is a Global scope, Function scope, and Block scope.
Global scope:
- Available outside of any function or block.
- Variables declared in Global scope are accessible everywhere.
const name = 'Dan';
const human = true;
Function scope:
- Variables are accessible only inside a function or block, NOT outside.
- It is also called Local scope.
function localScope() {
const localVariable = 'Local';
console.log(localVariable); // Accesses localVariable
}
localScope(); // Output: "Local"
console.log(localVariable); // Output: ReferenceError
Block scope:
- Variables are accessible only inside a block.
- #1 only applies to
let
andconst
variables. - Functions are also block scoped (only in Strict mode)
function localScope() {
const localVariable = 'Local';
console.log(localVariable);
}
localScope(); // Output: "Local"
console.log(localVariable); // Output: ReferenceError
Scope of a variable: The region of a code where a certain variable can be accessed.
Type Coercion
Type coercion is the process of converting one type of value into another. There are 3 types of conversion in JavaScript:
- to
string
- to
boolean
- to
number
let string = '1';
let number = 1;
number == string; // Output: true
// == is loose equality, === is strict equality
// double equals (==) will perform a type conversion
// one or both sides may undergo conversions
// in this case, 1 == 1 or '1' == '1' before checking equality
Strict equals: The triple equals (===) or strict equality compares two values without type coercion. If the values are not the same type, then the values are not equal.
let string = '1';
let number = 1;
number === string; // Output: false